:
| | | 6,216 | 05-05-2015, 11:40 PM : | | | | 2,662 | 10-19-2014, 09:04 PM : | | | | 3,717 | 03-06-2013, 03:26 AM : | | | | 5,142 | 09-12-2012, 08:57 PM : | - View a Printable Version
- Subscribe to this thread
- Learn QTP (UFT) Blog
- Privacy Policy
- Mobile Version
- RSS Syndication
- HTML Sitemap
- QTP / UFT Training
- UFT Certification Mock Papers

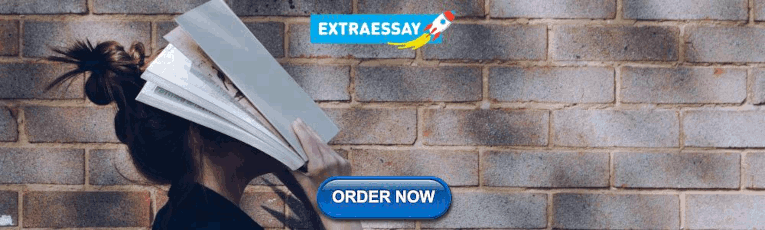
COMMENTS
Thanks for contributing an answer to Stack Overflow! Please be sure to answer the question.Provide details and share your research! But avoid …. Asking for help, clarification, or responding to other answers.
Visual Basic (Usage) C#. C++. J#. JScript. You invoked a procedure, but used either the wrong procedure name, the wrong number of arguments for this procedure, or wrong argument types. The number of arguments passed to a procedure must match the number of parameters in the procedure's definition.
UFT One User Discussions Home Discussions ... this does not work either. Then I get an error: 'Wrong number of arguments or invalid property assignment: 'Data Table' This is the script that I am using to enter information on a screen: ... Regarding descriptive programming, I am not entirely sure, but I assume that "index" is not a valid ...
Micro Focus QTP (UFT) Forums › Micro Focus UFT (earlier known as QTP) ... Wrong number of arguments has been pased or invalid property assignment. Can somebody tell whats wrong there[/align] Find. Reply. himabindhub Junior Member. Posts: 1 Threads: 0
Step3: Loop thru all the rows & retrieve required cell's data using 'GetCellData' method & Validate the current link text with expected link text. Step4 : If the required link text is found in 'i'th row, then use 'ChildItem' Method & select the correspoding CheckBox present in the same row & Exit out of the Loop.
I am having tough time figuring out what's wrong with the function call. I have a function which i am calling and the moment it enters the function i get the err.number = 501. I verified all the variables, all are string and it matches with the number of arguments defined in the function. No clue why am I getting this.
Errors: Wrong number of arguments or invalid property assignment can cause errors to occur. These errors can range from simple syntax errors to more serious runtime errors. Data corruption: Wrong number of arguments or invalid property assignment can also lead to data corruption. This can happen when a property is assigned to an object with the ...
If an answer to your question is correct, click on "Verify Answer" under the "More" button. The answer will now appear with a checkmark.
Hello, I've found this bit of code during my searches on the web and this does work for me in other spreadsheets however, when I try and run this in...
The main problem is that you named the macro Columns, which is also a keyword in Excel VBA. There is also a lot of superfluous code resulting from the recording process.
So I guess what I need to figure out is what property IS "Most Actives" When I use the object spy it says the WebTable: Most Actives, with a variety of attributes. Thats why I put the Most Actives in the webtable function in the first place.
I have a workbook that I use as a template to create other workbooks where the user collects information and sends that data to a data file. The main (starter) workbook is well used in many scenarios so I feel the VBA code is solid.
A vibrant community of Excel enthusiasts. Get expert tips, ask questions, and share your love for all things Excel. Elevate your spreadsheet skills with us!
Out of touch with vba and so i am sure its a silly mistake somewhere. Would be really helpful if someone could point that out. Code: Private Function generate() As Integer Dim source_size As Long Dim target_size As Long Dim i As Long Dim j As Long Dim count As Long Dim source1 As Range Dim target1 As Range Set source1 = Worksheets("Filter").Range(C4, C6498) Set target1 = Worksheets("30").Range ...
The button shows up fine. The procedure is in the same template as the ribbon modification. The onAction is "ContinuousPageNumbers1." When I click on the button I get the following error: If I go into the vba editor to the macro, I can run it fine. Same with running it directly in the template using the Macros dialog.
Use singleton instead of context property for settings. Commit. Port settings to FormCard. Commit. Move info button to the toolbar. Commit. Add titles for breathing pages. Commit. Port away from OverlaySheet. Commit. Run latest qmlformat. Commit. Don't use context property to expose AboutData to qml. Commit. Flatpak: update Kirigami Addons to 1 ...
I'm writing this script to help with logging when my workplace re-images computers. The problem is that I am somewhat inexperienced with VBS and troubleshooting VBS scripts.
Wrong number of arguments or invalid property assignment. ... Thread Modes. Wrong number of arguments or invalid property assignment. qtpro_exe Junior Member. Posts: 1 Threads: 1 Joined: Nov 2011 Reputation: 0 #1. 11-22-2011, 07:48 AM . I am facing an issue while clicking the filter button during automation.Using QTP 11 and IE 7. I am getting ...