- Table of Contents
- Scratch ActiveCode
- Navigation Help
- Help for Instructors
- About Runestone
- Report A Problem
- 1. Introduction
- 2. Analysis
- 3. Basic Data Structures
- 4. Recursion
- 5. Sorting and Searching
- 6. Trees and Tree Algorithms
- 7. Graphs and Graph Algorithms
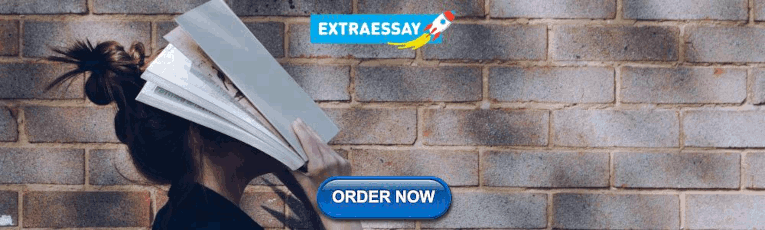
Problem Solving with Algorithms and Data Structures using Python ¶
By Brad Miller and David Ranum, Luther College (as remixed by Jeffrey Elkner)
- 1.1. Objectives
- 1.2. Getting Started
- 1.3. What Is Computer Science?
- 1.4. What Is Programming?
- 1.5. Why Study Data Structures and Abstract Data Types?
- 1.6. Why Study Algorithms?
- 1.7. Review of Basic Python
- 1.8.1. Built-in Atomic Data Types
- 1.8.2. Built-in Collection Data Types
- 1.9.1. String Formatting
- 1.10. Control Structures
- 1.11. Exception Handling
- 1.12. Defining Functions
- 1.13.1. A Fraction Class
- 1.13.2. Inheritance: Logic Gates and Circuits
- 1.14. Summary
- 1.15. Key Terms
- 1.16. Discussion Questions
- 1.17. Programming Exercises
- 2.1. Objectives
- 2.2. What Is Algorithm Analysis?
- 2.3. Big-O Notation
- 2.4.1. Solution 1: Checking Off
- 2.4.2. Solution 2: Sort and Compare
- 2.4.3. Solution 3: Brute Force
- 2.4.4. Solution 4: Count and Compare
- 2.5. Performance of Python Data Structures
- 2.7. Dictionaries
- 2.8. Summary
- 2.9. Key Terms
- 2.10. Discussion Questions
- 2.11. Programming Exercises
- 3.1. Objectives
- 3.2. What Are Linear Structures?
- 3.3. What is a Stack?
- 3.4. The Stack Abstract Data Type
- 3.5. Implementing a Stack in Python
- 3.6. Simple Balanced Parentheses
- 3.7. Balanced Symbols (A General Case)
- 3.8. Converting Decimal Numbers to Binary Numbers
- 3.9.1. Conversion of Infix Expressions to Prefix and Postfix
- 3.9.2. General Infix-to-Postfix Conversion
- 3.9.3. Postfix Evaluation
- 3.10. What Is a Queue?
- 3.11. The Queue Abstract Data Type
- 3.12. Implementing a Queue in Python
- 3.13. Simulation: Hot Potato
- 3.14.1. Main Simulation Steps
- 3.14.2. Python Implementation
- 3.14.3. Discussion
- 3.15. What Is a Deque?
- 3.16. The Deque Abstract Data Type
- 3.17. Implementing a Deque in Python
- 3.18. Palindrome-Checker
- 3.19. Lists
- 3.20. The Unordered List Abstract Data Type
- 3.21.1. The Node Class
- 3.21.2. The Unordered List Class
- 3.22. The Ordered List Abstract Data Type
- 3.23.1. Analysis of Linked Lists
- 3.24. Summary
- 3.25. Key Terms
- 3.26. Discussion Questions
- 3.27. Programming Exercises
- 4.1. Objectives
- 4.2. What Is Recursion?
- 4.3. Calculating the Sum of a List of Numbers
- 4.4. The Three Laws of Recursion
- 4.5. Converting an Integer to a String in Any Base
- 4.6. Stack Frames: Implementing Recursion
- 4.7. Introduction: Visualizing Recursion
- 4.8. Sierpinski Triangle
- 4.9. Complex Recursive Problems
- 4.10. Tower of Hanoi
- 4.11. Exploring a Maze
- 4.12. Dynamic Programming
- 4.13. Summary
- 4.14. Key Terms
- 4.15. Discussion Questions
- 4.16. Glossary
- 4.17. Programming Exercises
- 5.1. Objectives
- 5.2. Searching
- 5.3.1. Analysis of Sequential Search
- 5.4.1. Analysis of Binary Search
- 5.5.1. Hash Functions
- 5.5.2. Collision Resolution
- 5.5.3. Implementing the Map Abstract Data Type
- 5.5.4. Analysis of Hashing
- 5.6. Sorting
- 5.7. The Bubble Sort
- 5.8. The Selection Sort
- 5.9. The Insertion Sort
- 5.10. The Shell Sort
- 5.11. The Merge Sort
- 5.12. The Quick Sort
- 5.13. Summary
- 5.14. Key Terms
- 5.15. Discussion Questions
- 5.16. Programming Exercises
- 6.1. Objectives
- 6.2. Examples of Trees
- 6.3. Vocabulary and Definitions
- 6.4. List of Lists Representation
- 6.5. Nodes and References
- 6.6. Parse Tree
- 6.7. Tree Traversals
- 6.8. Priority Queues with Binary Heaps
- 6.9. Binary Heap Operations
- 6.10.1. The Structure Property
- 6.10.2. The Heap Order Property
- 6.10.3. Heap Operations
- 6.11. Binary Search Trees
- 6.12. Search Tree Operations
- 6.13. Search Tree Implementation
- 6.14. Search Tree Analysis
- 6.15. Balanced Binary Search Trees
- 6.16. AVL Tree Performance
- 6.17. AVL Tree Implementation
- 6.18. Summary of Map ADT Implementations
- 6.19. Summary
- 6.20. Key Terms
- 6.21. Discussion Questions
- 6.22. Programming Exercises
- 7.1. Objectives
- 7.2. Vocabulary and Definitions
- 7.3. The Graph Abstract Data Type
- 7.4. An Adjacency Matrix
- 7.5. An Adjacency List
- 7.6. Implementation
- 7.7. The Word Ladder Problem
- 7.8. Building the Word Ladder Graph
- 7.9. Implementing Breadth First Search
- 7.10. Breadth First Search Analysis
- 7.11. The Knight’s Tour Problem
- 7.12. Building the Knight’s Tour Graph
- 7.13. Implementing Knight’s Tour
- 7.14. Knight’s Tour Analysis
- 7.15. General Depth First Search
- 7.16. Depth First Search Analysis
- 7.17. Topological Sorting
- 7.18. Strongly Connected Components
- 7.19. Shortest Path Problems
- 7.20. Dijkstra’s Algorithm
- 7.21. Analysis of Dijkstra’s Algorithm
- 7.22. Prim’s Spanning Tree Algorithm
- 7.23. Summary
- 7.24. Key Terms
- 7.25. Discussion Questions
- 7.26. Programming Exercises
Acknowledgements ¶
We are very grateful to Franklin Beedle Publishers for allowing us to make this interactive textbook freely available. This online version is dedicated to the memory of our first editor, Jim Leisy, who wanted us to “change the world.”
Indices and tables ¶
- Module Index
- Search Page

- Corpus ID: 60148039
Problem solving with algorithms and data structures using Python
- Bradley N. Miller , D. Ranum
- Published 1 September 2005
- Computer Science
17 Citations
Teaching an object-oriented cs1 -: with python, using python to teach object-oriented programming in cs1, experience: from c++ to python in 3 easy steps, teaching an object-oriented cs 1 in python, tuk tuk: a block-based programming game, putting in all the stops: execution control for javascript, solving scheduling problems with randomized and parallelized brute-force approach, new and improved search algorithms and precise analysis of their average-case complexity, building an interactive robotics control laboratory with python, identifying cleartext in historical ciphers, related papers.
Showing 1 through 3 of 0 Related Papers

- Runestone in social media: Follow @iRunestone Our Facebook Page
- Table of Contents
- Assignments
- Peer Instruction (Instructor)
- Peer Instruction (Student)
- Change Course
- Instructor's Page
- Progress Page
- Edit Profile
- Change Password
- Scratch ActiveCode
- Scratch Activecode
- Instructors Guide
- About Runestone
- Report A Problem
- This Chapter
- 1. Introduction' data-toggle="tooltip" >
Problem Solving with Algorithms and Data Structures using Python ¶

By Brad Miller and David Ranum, Luther College
There is a wonderful collection of YouTube videos recorded by Gerry Jenkins to support all of the chapters in this text.
- 1.1. Objectives
- 1.2. Getting Started
- 1.3. What Is Computer Science?
- 1.4. What Is Programming?
- 1.5. Why Study Data Structures and Abstract Data Types?
- 1.6. Why Study Algorithms?
- 1.7. Review of Basic Python
- 1.8.1. Built-in Atomic Data Types
- 1.8.2. Built-in Collection Data Types
- 1.9.1. String Formatting
- 1.10. Control Structures
- 1.11. Exception Handling
- 1.12. Defining Functions
- 1.13.1. A Fraction Class
- 1.13.2. Inheritance: Logic Gates and Circuits
- 1.14. Summary
- 1.15. Key Terms
- 1.16. Discussion Questions
- 1.17. Programming Exercises
- 2.1.1. A Basic implementation of the MSDie class
- 2.2. Making your Class Comparable
- 3.1. Objectives
- 3.2. What Is Algorithm Analysis?
- 3.3. Big-O Notation
- 3.4.1. Solution 1: Checking Off
- 3.4.2. Solution 2: Sort and Compare
- 3.4.3. Solution 3: Brute Force
- 3.4.4. Solution 4: Count and Compare
- 3.5. Performance of Python Data Structures
- 3.7. Dictionaries
- 3.8. Summary
- 3.9. Key Terms
- 3.10. Discussion Questions
- 3.11. Programming Exercises
- 4.1. Objectives
- 4.2. What Are Linear Structures?
- 4.3. What is a Stack?
- 4.4. The Stack Abstract Data Type
- 4.5. Implementing a Stack in Python
- 4.6. Simple Balanced Parentheses
- 4.7. Balanced Symbols (A General Case)
- 4.8. Converting Decimal Numbers to Binary Numbers
- 4.9.1. Conversion of Infix Expressions to Prefix and Postfix
- 4.9.2. General Infix-to-Postfix Conversion
- 4.9.3. Postfix Evaluation
- 4.10. What Is a Queue?
- 4.11. The Queue Abstract Data Type
- 4.12. Implementing a Queue in Python
- 4.13. Simulation: Hot Potato
- 4.14.1. Main Simulation Steps
- 4.14.2. Python Implementation
- 4.14.3. Discussion
- 4.15. What Is a Deque?
- 4.16. The Deque Abstract Data Type
- 4.17. Implementing a Deque in Python
- 4.18. Palindrome-Checker
- 4.19. Lists
- 4.20. The Unordered List Abstract Data Type
- 4.21.1. The Node Class
- 4.21.2. The Unordered List Class
- 4.22. The Ordered List Abstract Data Type
- 4.23.1. Analysis of Linked Lists
- 4.24. Summary
- 4.25. Key Terms
- 4.26. Discussion Questions
- 4.27. Programming Exercises
- 5.1. Objectives
- 5.2. What Is Recursion?
- 5.3. Calculating the Sum of a List of Numbers
- 5.4. The Three Laws of Recursion
- 5.5. Converting an Integer to a String in Any Base
- 5.6. Stack Frames: Implementing Recursion
- 5.7. Introduction: Visualizing Recursion
- 5.8. Sierpinski Triangle
- 5.9. Complex Recursive Problems
- 5.10. Tower of Hanoi
- 5.11. Exploring a Maze
- 5.12. Dynamic Programming
- 5.13. Summary
- 5.14. Key Terms
- 5.15. Discussion Questions
- 5.16. Glossary
- 5.17. Programming Exercises
- 6.1. Objectives
- 6.2. Searching
- 6.3.1. Analysis of Sequential Search
- 6.4.1. Analysis of Binary Search
- 6.5.1. Hash Functions
- 6.5.2. Collision Resolution
- 6.5.3. Implementing the Map Abstract Data Type
- 6.5.4. Analysis of Hashing
- 6.6. Sorting
- 6.7. The Bubble Sort
- 6.8. The Selection Sort
- 6.9. The Insertion Sort
- 6.10. The Shell Sort
- 6.11. The Merge Sort
- 6.12. The Quick Sort
- 6.13. Summary
- 6.14. Key Terms
- 6.15. Discussion Questions
- 6.16. Programming Exercises
- 7.1. Objectives
- 7.2. Examples of Trees
- 7.3. Vocabulary and Definitions
- 7.4. List of Lists Representation
- 7.5. Nodes and References
- 7.6. Parse Tree
- 7.7. Tree Traversals
- 7.8. Priority Queues with Binary Heaps
- 7.9. Binary Heap Operations
- 7.10.1. The Structure Property
- 7.10.2. The Heap Order Property
- 7.10.3. Heap Operations
- 7.11. Binary Search Trees
- 7.12. Search Tree Operations
- 7.13. Search Tree Implementation
- 7.14. Search Tree Analysis
- 7.15. Balanced Binary Search Trees
- 7.16. AVL Tree Performance
- 7.17. AVL Tree Implementation
- 7.18. Summary of Map ADT Implementations
- 7.19. Summary
- 7.20. Key Terms
- 7.21. Discussion Questions
- 7.22. Programming Exercises
- 8.1. Objectives
- 8.2. Vocabulary and Definitions
- 8.3. The Graph Abstract Data Type
- 8.4. An Adjacency Matrix
- 8.5. An Adjacency List
- 8.6. Implementation
- 8.7. The Word Ladder Problem
- 8.8. Building the Word Ladder Graph
- 8.9. Implementing Breadth First Search
- 8.10. Breadth First Search Analysis
- 8.11. The Knight’s Tour Problem
- 8.12. Building the Knight’s Tour Graph
- 8.13. Implementing Knight’s Tour
- 8.14. Knight’s Tour Analysis
- 8.15. General Depth First Search
- 8.16. Depth First Search Analysis
- 8.17. Topological Sorting
- 8.18. Strongly Connected Components
- 8.19. Shortest Path Problems
- 8.20. Dijkstra’s Algorithm
- 8.21. Analysis of Dijkstra’s Algorithm
- 8.22. Prim’s Spanning Tree Algorithm
- 8.23. Summary
- 8.24. Key Terms
- 8.25. Discussion Questions
- 8.26. Programming Exercises
Acknowledgements ¶
We are very grateful to Franklin Beedle Publishers for allowing us to make this interactive textbook freely available. This online version is dedicated to the memory of our first editor, Jim Leisy, who wanted us to “change the world.”
404 - NotFound
The resource you have requested cannot be found.
We're sorry :-(
- Computers & Technology
- Programming
Sorry, there was a problem.

Download the free Kindle app and start reading Kindle books instantly on your smartphone, tablet, or computer - no Kindle device required .
Read instantly on your browser with Kindle for Web.
Using your mobile phone camera - scan the code below and download the Kindle app.

Image Unavailable

- To view this video download Flash Player

Follow the author

Problem Solving with Algorithms and Data Structures Using Python 2nd Edition 2nd Edition
- ISBN-10 1590282574
- ISBN-13 978-1590282571
- Edition 2nd
- Publisher Franklin, Beedle & Associates
- Publication date August 22, 2011
- Language English
- Dimensions 7.5 x 0.99 x 9.25 inches
- Print length 438 pages
- See all details
Customers who bought this item also bought

Product details
- Publisher : Franklin, Beedle & Associates; 2nd edition (August 22, 2011)
- Language : English
- Paperback : 438 pages
- ISBN-10 : 1590282574
- ISBN-13 : 978-1590282571
- Item Weight : 1.7 pounds
- Dimensions : 7.5 x 0.99 x 9.25 inches
- #18 in Data Structure and Algorithms
- #505 in Microsoft Programming (Books)
- #524 in Python Programming
About the author
Bradley n. miller.
Discover more of the author’s books, see similar authors, read author blogs and more
Customer reviews
- 5 star 4 star 3 star 2 star 1 star 5 star 75% 17% 4% 1% 3% 75%
- 5 star 4 star 3 star 2 star 1 star 4 star 75% 17% 4% 1% 3% 17%
- 5 star 4 star 3 star 2 star 1 star 3 star 75% 17% 4% 1% 3% 4%
- 5 star 4 star 3 star 2 star 1 star 2 star 75% 17% 4% 1% 3% 1%
- 5 star 4 star 3 star 2 star 1 star 1 star 75% 17% 4% 1% 3% 3%
Customer Reviews, including Product Star Ratings help customers to learn more about the product and decide whether it is the right product for them.
To calculate the overall star rating and percentage breakdown by star, we don’t use a simple average. Instead, our system considers things like how recent a review is and if the reviewer bought the item on Amazon. It also analyzed reviews to verify trustworthiness.
Reviews with images

- Sort reviews by Top reviews Most recent Top reviews
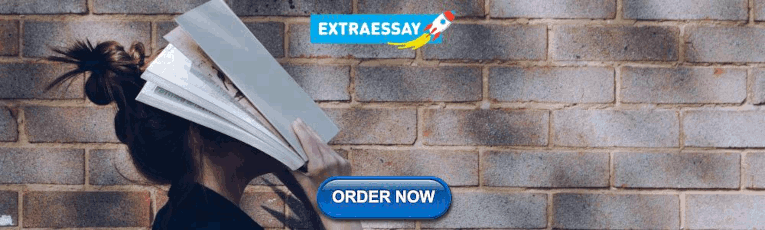
Top reviews from the United States
There was a problem filtering reviews right now. please try again later..

Top reviews from other countries

- About Amazon
- Investor Relations
- Amazon Devices
- Amazon Science
- Sell products on Amazon
- Sell on Amazon Business
- Sell apps on Amazon
- Become an Affiliate
- Advertise Your Products
- Self-Publish with Us
- Host an Amazon Hub
- › See More Make Money with Us
- Amazon Business Card
- Shop with Points
- Reload Your Balance
- Amazon Currency Converter
- Amazon and COVID-19
- Your Account
- Your Orders
- Shipping Rates & Policies
- Returns & Replacements
- Manage Your Content and Devices
- Conditions of Use
- Privacy Notice
- Consumer Health Data Privacy Disclosure
- Your Ads Privacy Choices
Academia.edu no longer supports Internet Explorer.
To browse Academia.edu and the wider internet faster and more securely, please take a few seconds to upgrade your browser .
Enter the email address you signed up with and we'll email you a reset link.
- We're Hiring!
- Help Center

Data Structures and Algorithms with Python - Kent D. Lee & Steve Hubbard

Undergraduate Topics in Computer Science (UTiCS) delivers high-quality instructional content for undergraduates studying in all areas of computing and information science. From core foundational and theoretical material to final-year topics and applications, UTiCS books take a fresh, concise, and modern approach and are ideal for self-study or for a one- or two-semester course. The texts are all authored by established experts in their fields, reviewed by an international advisory board, and contain numerous examples and problems. Many include fully worked solutions. More information about this series at http://www.springer.com/series/7592
Related Papers
Ashutosh Prakash

A.R.S. Publications, Chennai
P Krishna Sankar
(CD3291 – Data Structures and Algorithms & CD3281 – Data Structures and Algorithm Laboratory - B. Tech. – Information Technology - As per the Latest Syllabus of Anna University, Chennai - Regulation 2021) This book "Data Structures and Algorithms" is about basic idea towards data representation in program and its manipulation. It provides a clear view towards Abstract Data Type and Object-Oriented Programming on Python. It provides a preliminary study on linear data structures, sorting, searching, hashing, Tree and Graph Structures along with Python implementation. Unit I: Introduction towards Abstract Data Types and Object-Oriented Programming. Contributes a knowledge on analysis of algorithm, asymptotic notations, divide & conquer and recursion with example. Unit II: Summary on Linear structures and its working mechanism. Provides an hands on understanding towards the Array List, Linked List, Stack and Queue. Linked list were represented with singly, doubly, circularly, stack and queue through Python. Unit III: Brief knowledge over sorting and searching. Bubble, Selection, Insertion, Merge, Quick sort implemented through Python. It provides detailed understanding and procedures for linear search, binary search, hash functions and collision handling. Unit IV: Transitory awareness on Tree and its traversal. Provides a procedure in Python to construct Binary Tree, AVL Tree, Heap, B Tree & B+ Tree and Tree Traversal. Unit V: Provides a study over graph and its traversal mechanisms. Python hands on experience over estimating shortest path and constructing minimum spanning tree over a graph. Understanding towards problem complexity and its classes. Unit VI: It provides an implementation idea over recursive algorithm, List, Stack and Queue. Understanding towards the several sorting and searching algorithm using python. Detailed implementation to construct tree traversal, minimum spanning tree and estimate the shortest path on graph through Python.
Nakul Singh
JEANBAPTISTE MBANZABUGABO
DATA STRUCTURES AND ALGORITHMS - STUDENT VERSION UNIVERSITY OF TOURISM TECHNOLOGY AND BUSINESS STUDIES(UTB) – DEPARTMENT OF BUSINESS INFORMATION TECHNOLOGY “Just-in-time-erudition, learning by case in point, and Learning by doing and Demonstrating.”
Jose Luis Garavito Alejos
Camilo Vargas
users.utcluj.ro
Marius Joldos
Anjo Tadena
Jestin Mathew
With a dynamic learn-by-doing focus, this document encourages students to explore data structures by implementing them, a process through which students discover how data structures work and how they can be applied. Providing a framework that offers feedback and support, this text challenges students to exercise their creativity in both programming and analysis. Each laboratory work creates an excellent hands-on learning opportunity for students. Students will be expected to write C-language programs, ranging from very short programs to more elaborate systems. Since one of the goals of this course is to teach how to write large, reliable programs. We will be emphasizing the development of clear, modular programs that are easy to read, debug, verify, analyze, and modify. PREREQUISITE: A good knowledge of c-language, use of Function and structures.
Loading Preview
Sorry, preview is currently unavailable. You can download the paper by clicking the button above.
RELATED PAPERS
Siddhartha Mickey
Kavya Mishra
tuyệt thiên vô tình
Okoro U Raymond , Afolashade Kuyoro
CH V GANESH
Larry Denenberg
Nada Shaban
Sagar Bommidi
M. Liebmann , Gundolf Haase
Lara Alnajjar
Isromi Janwar
Leah Ellacott
manshu agrawal
ALI MOULAEI NEJAD
Islamic Story
Saiful Islam
Shyam Sapkota
Operations Research/Computer Science Interfaces Series
Robert Tarjan
Nsubuga Herbert Chris
srikrishna d
Priyank Paliwal
Cliff Shaffer
RELATED TOPICS
- We're Hiring!
- Help Center
- Find new research papers in:
- Health Sciences
- Earth Sciences
- Cognitive Science
- Mathematics
- Computer Science
- Academia ©2024
- Skip to right header navigation
- Skip to main content
- Skip to secondary navigation
- Skip to primary sidebar
Legally Free Computer Books
- All Categories
- Privacy policy
Problem Solving with Algorithms and Data Structures Using Python
October 14, 2012
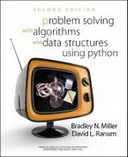
“Problem Solving with Algorithms and Data Structures Using Python” , by Brad Miller and David Ranum, is an interactive book which you can access online.
Book Description
Computer science is the study of problems, problem-solving, and the solutions that come out of the problem-solving process. Given a problem, a computer scientist’s goal is to develop an algorithm, a step-by-step list of instructions for solving any instance of the problem that might arise. Algorithms are finite processes that if followed will solve the problem. Algorithms are solutions.
Table of Contents
- Introduction
- Basic Data Structures
- Sorting and Searching
- Trees and Tree Agorithms
- Graphs and Graph Algorithms
Download Free PDF / Read Online
Similar books:.
- Data Structures and Algorithm Analysis in C++
- Data Structures and Algorithm Analysis in Java
- Dictionary of Algorithms and Data Structures
- Sorting and Searching Algorithms: A Cookbook
- Java Data Structures (2nd edition)
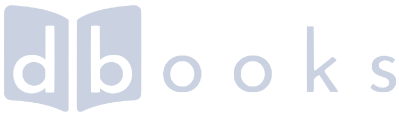
Problem Solving with Algorithms and Data Structures
by Brad Miller, David Ranum
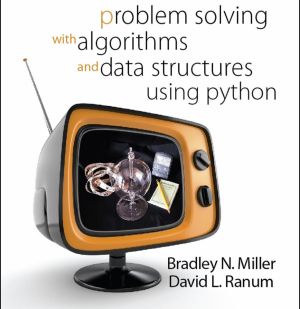
Subscribe to new books via dBooks.org telegram channel
Book description.
This open book is licensed under a Creative Commons License (CC BY). You can download Problem Solving with Algorithms and Data Structures ebook for free in PDF format (4.9 MB).
Table of Contents
Book details.

Book Hashtags
Related books.
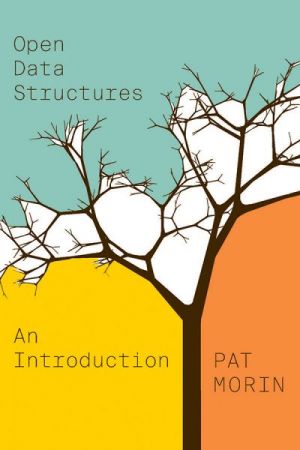
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
Hemant-Jain-Author/Problem-Solving-in-Data-Structures-Algorithms-using-Python
Folders and files.
Name | Name | |||
---|---|---|---|---|
53 Commits | ||||
Repository files navigation
Problem-solving-in-data-structures-algorithms-using-python3.
This is the code repository of book Problem Solving in Data Structures & Algorithms using Python .
About The Book
- This textbook provides in depth coverage of various Data Structures and Algorithms.
- Concepts are discussed in easy to understand manner.
- Large number of diagrams are provided to grasp concepts easily.
- Time and Space complexities of various algorithms are discussed.
- Helpful for interviews preparation and competitive coding.
- Large number of interview questions are solved.
- Python solutions are provided with input and output.
- Guide you through how to solve new problems in programming interview of various software companies.
Table of Contents
- Chapter 0: How to use this book.
- Chapter 1: Algorithms Analysis
- Chapter 2: Approach to solve algorithm design problems
- Chapter 3: Abstract Data Type & Python Collections
- Chapter 4: Searching
- Chapter 5: Sorting
- Chapter 6: Linked List
- Chapter 7: Stack
- Chapter 8: Queue
- Chapter 9: Tree
- Chapter 10: Priority Queue
- Chapter 11: Hash-Table
- Chapter 12: Graphs
- Chapter 13: String Algorithms
- Chapter 14: Algorithm Design Techniques
- Chapter 15: Brute Force Algorithm
- Chapter 16: Greedy Algorithm
- Chapter 17: Divide & Conquer
- Chapter 18: Dynamic Programming
- Chapter 19: Backtracking
- Chapter 20: Complexity Theory
- Python 100.0%
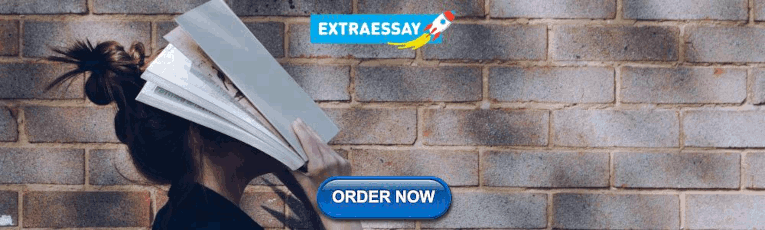
IMAGES
COMMENTS
An interactive version of Problem Solving with Algorithms and Data Structures using Python. ... Problem Solving with Algorithms and Data Structures using Python by Bradley N. Miller, David L. Ranum is licensed under a Creative Commons Attribution-NonCommercial-ShareAlike 4.0 International License.
Use saved searches to filter your results more quickly. Name. Query. To see all available qualifiers, see our documentation. Cancel Create saved search Sign in Sign up Reseting focus. You signed in with another tab or window. Reload to refresh your session. You signed out in another tab or window. ...
Problem solving with algorithms and data structures using Python. Bradley N. Miller, D. Ranum. Published 1 September 2005. Computer Science. TLDR. This textbook is designed to serve as a text for a first course on data structures and algorithms, typically taught as the second course in the computer science curriculum, and assumes beginners at ...
Problem Solving with Algorithms and Data Structures using Python¶. By Brad Miller and David Ranum, Luther College. Assignments; There is a wonderful collection of YouTube videos recorded by Gerry Jenkins to support all of the chapters in this text.
We cover abstract data types and data structures, writing algorithms, and solving problems. We look at a number of data structures and solve classic problems that arise. The tools and techniques that you learn here will be applied over and over as you continue your study of computer science. About the Authors. N/A; Reviews, Ratings, and ...
students can understand the concept easily. All the relevant data structures and their operations are discussed with dia. rams and examples for better understanding. After discussing relevant algorithms in detail, the algorithmic representation and. the corresponding code in Python are given.
Problem Solving with Algorithms and Data Structures Using Python Bradley N. Miller,David L. Ranum,Roman Yasinovskyy,2023 This textbook uses Python language and is designed to serve as a text for a first course on data structures and algorithms, typically taught as the second course in the college-level computer science curriculum.
Contents 1 Introduction 1 1.1 Modern Computers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .1 1.2 Computer Languages ...
THIS TEXTBOOK is about computer science. It is also about Python. However, there is much more. The study of algorithms and data structures is central to understanding what computer science is all about. Learning computer science is not unlike learning any other type of difficult subject matter. The only way to be successful is through deliberate and incremental exposure to the fundamental ideas.
Problem Solving With Algorithms AndAn algorithm is a plan for solving a problem. A person must design an algorithm. A person must translate an algorithm into a computer program. This point of view sets the stage for a process that we will use to develop solutions to Jeroo problems.4.
You signed in with another tab or window. Reload to refresh your session. You signed out in another tab or window. Reload to refresh your session. You switched accounts on another tab or window.
Problem Solving with Algorithms and Data Structures in python byBrad Miller, David Ranum. ... Problem Solving with Algorithms and Data Structures in python by Brad Miller, David Ranum. ... 2023-08-10 07:26:24 Identifier problem-solvingwith-algorithmsand-data-structures Identifier-ark ark:/13960/s2nc45sxtkh Ocr tesseract 5.3.0-3-g9920
Problem Solving with Algorithms and Data Structures using Python by Brad Miller and David Ranum is licensed under a Creative Commons Attribution-NonCommercial-ShareAlike 4.0 International License. About
Data Structures and Algorithms in Python provides an introduction to data structures and algorithms, including their design, analysis, and implementation. This book is designed for use in a beginning-level data structures course, or in an intermediate-level introduction to algorithms course.
Problem Solving in Data Structures & Algorithms Using Python Hemant Jain,2019-05-16 Problem Solving in Data Structures & Algorithms is a series of books about the usage of Data Structures and Algorithms in computer programming. The book is easy to follow and is written for interview preparation point of view.
We cover abstract data types and data structures, writing algorithms, and solving problems. We look at a number of data structures and solve classic problems that arise. The tools and techniques that you learn here will be applied over and over as you continue your study of computer science.
Solutions to self-checks and exercises from the book, "Problem Solving with Algorithms and Data Structures Using Python" by Brad Miller and David Ranum - paxcodes/book_pythonds3 ... "Problem Solving with Algorithms and Data Structures Using Python" by Brad Miller and David Ranum Resources. Readme Activity. Stars. 0 stars Watchers. 2 watching Forks.
Python hands on experience over estimating shortest path and constructing minimum spanning tree over a graph. Understanding towards problem complexity and its classes. Unit VI: It provides an implementation idea over recursive algorithm, List, Stack and Queue. Understanding towards the several sorting and searching algorithm using python.
Problem Solving with Algorithms and Data Structures, Release 3.0 Control constructs allow algorithmic steps to be represented in a convenient yet unambiguous way. At a minimum, algorithms require constructs that perform sequential processing, selection for decision-making, and iteration for repetitive control. As long as the language provides these
"Problem Solving with Algorithms and Data Structures Using Python", by Brad Miller and David Ranum, is an interactive book which you can access online. Book Description. Computer science is the study of problems, problem-solving, and the solutions that come out of the problem-solving process.
jacobwis/problem-solving-with-algorithms-and-data-structures-using-python This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository. master
We cover abstract data types and data structures, writing algorithms, and solving problems. We look at a number of data structures and solve classic problems that arise. The tools and techniques that you learn here will be applied over and over as you continue your study of computer science.
Problem-Solving-in-Data-Structures-Algorithms-using-Python3. This is the code repository of book Problem Solving in Data Structures & Algorithms using Python. About The Book. This textbook provides in depth coverage of various Data Structures and Algorithms. Concepts are discussed in easy to understand manner.